Deep Dive into Software Testing: Handling Comment Edits
Hey LinkedIn Learners,
Today, I'd like to share a snippet from one of my software tests, written in Jest for a React/Next.js app. While we often talk about the importance of building robust features, it's equally crucial to ensure those features work flawlessly under various scenarios. Let's delve into one such test!
The Setup
We've got a post with comments, and users should be able to edit their own comments. We start with a mock comment and aim to modify it.
const specialComments = [{ ... text: 'old comment' }];
The Sequence
1. Simulate user interaction to expand a post.
2. Open the menu that is attached to the user's comment.
fireEvent.click(getByLabelText('Open menu'));
fireEvent.click(getByText('Edit'));
3. Edit the comment content.
const input = getByLabelText('Edit Comment Input');
userEvent.clear(input);
await userEvent.type(input, 'This is an edited comment');
4. Submit the changes.
The Magic
- For UI interactions, we leverage both fireEvent and userEvent functions.
- For typing in inputs, we prefer userEvent over a simple fireEvent. Why? Because userEvent.type closely mimics human behavior by focusing on the input, and simulating the keypress of each individual character, offering a more realistic interaction for our tests.
- Given the async nature of user events, we deploy async/await to handle these gracefully.
The Validation
Post-edit, our test checks if the comment's content has been updated in the UI. The expectation? To find the revised comment - 'This is an edited comment'.
The importance of such tests? Ensuring that our users experience a seamless and bug-free environment while interacting with the app.
Check out the full test.
Would love to hear your thoughts or any tips on enhancing software testing!
More
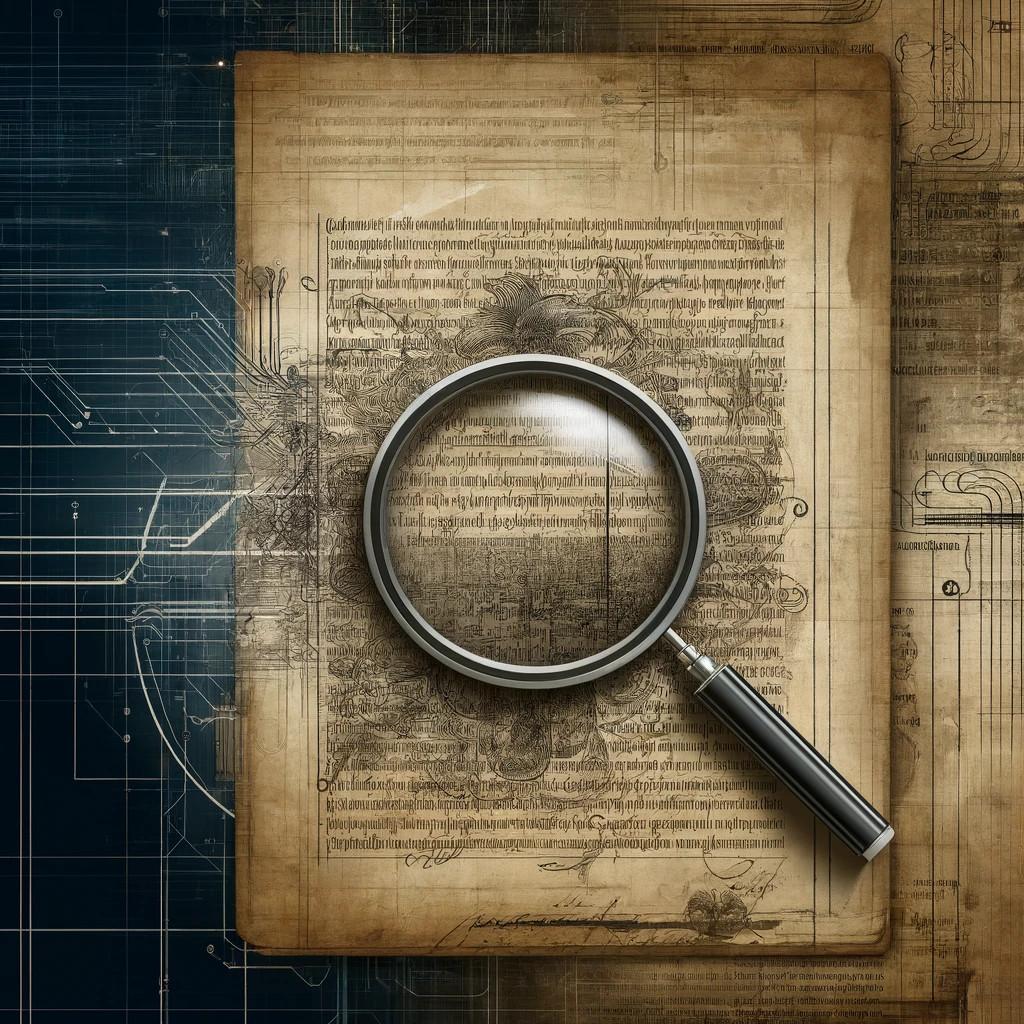
Deciphering the Past: A Journey of Transcribing Handwritten Journals
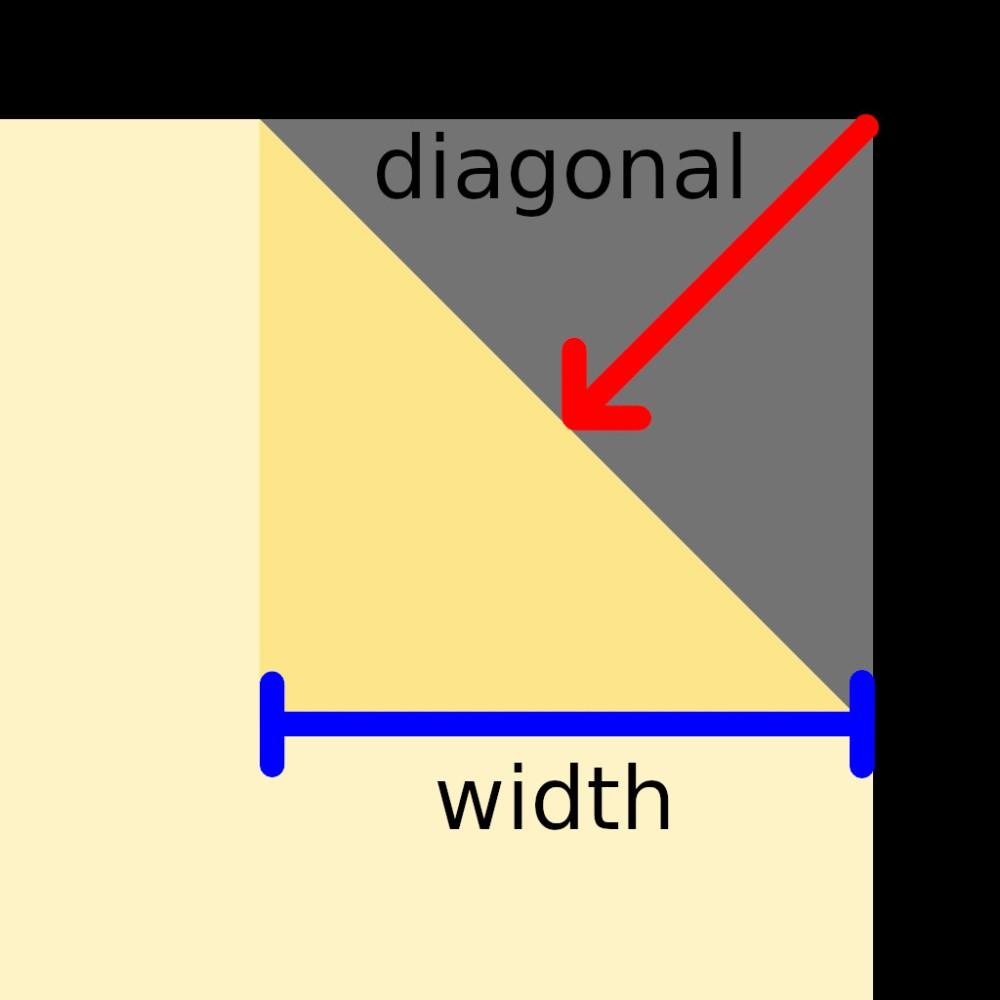
Turn the Corner on Your Web Design Skills with This CSS Page Fold Trick
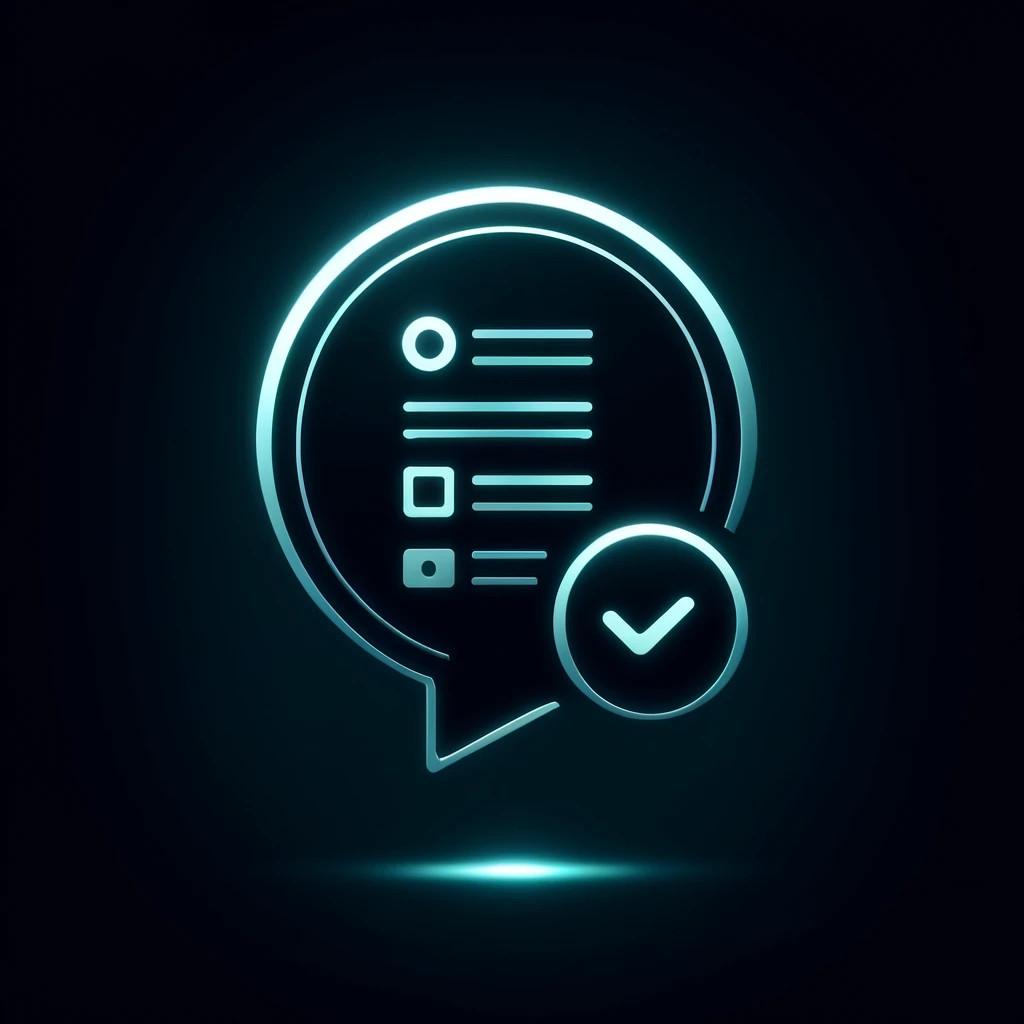
Theme Switching with Next.js and DaisyUI - Light and Dark Modes
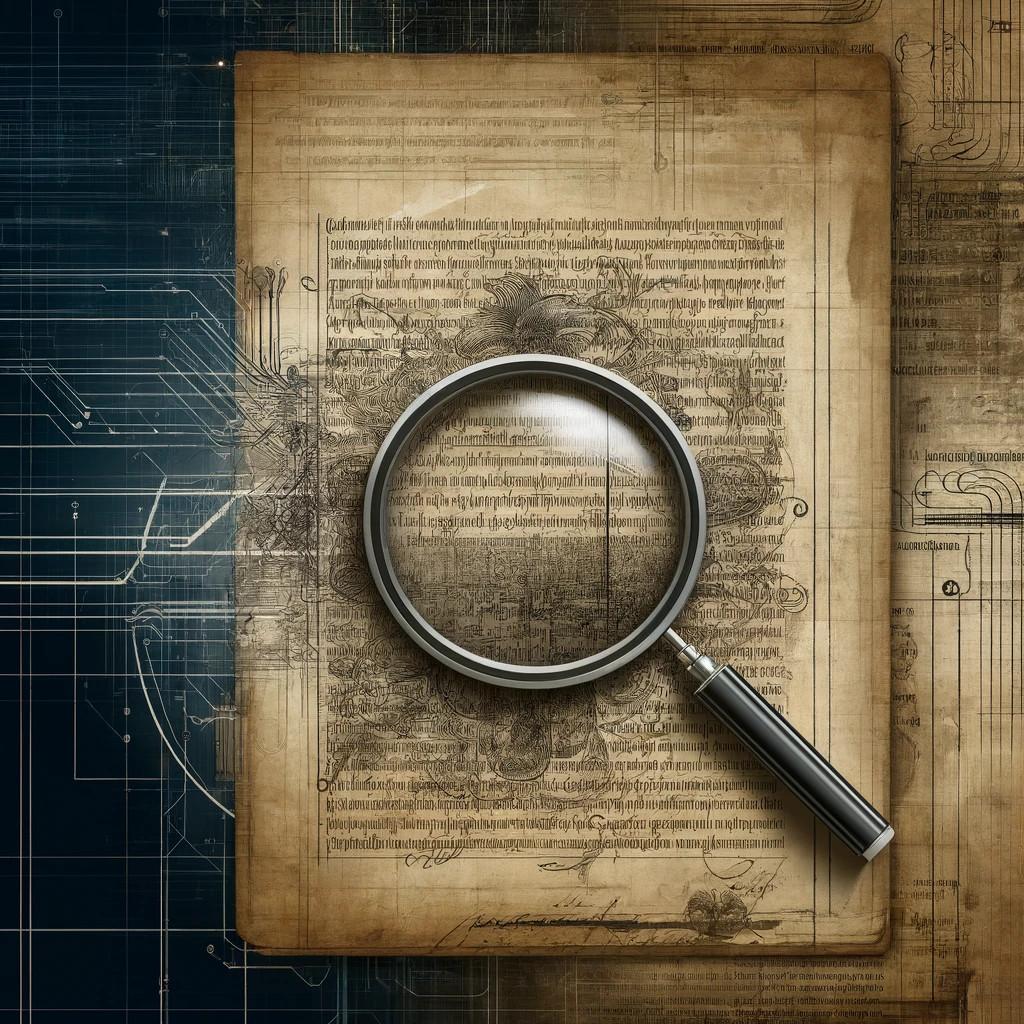